React 17 and newer
Step 1: Install LibraryCopied!
Install the Rollout Link library in your React app:
npm install @rollout/link-react
Step 2: Render componentCopied!
import React, { useState, useEffect } from "react";
import { RolloutLinkProvider, CredentialsManager } from "@rollout/link-react";
function fetchRolloutToken() {
// We generate the token on the server in order to keep
// the ROLLOUT_CLIENT_SECRET secure.
return fetch("/rollout-token")
.then((resp) => resp.json())
.then((data) => data.token);
}
export function App() {
return (
<div id="app">
<RolloutLinkProvider token={fetchRolloutToken}>
<CredentialsManager />
</RolloutLinkProvider>
</div>
);
}
Now you should see something that looks like this:
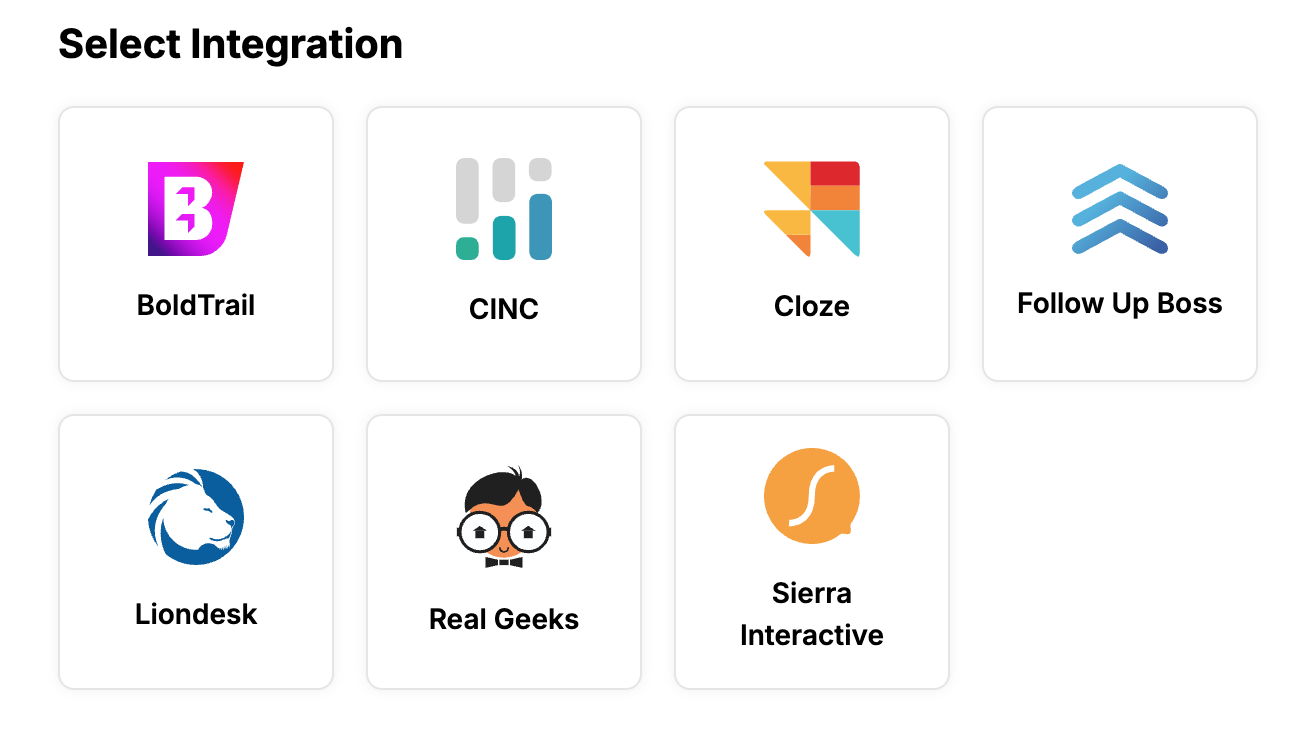
Step 3 (Optional): Handle when new credentials is addedCopied!
When a user authenticates with a new credential, you can call a function to handle that interaction. Whether you want to display a new page or store that credential in your database (you can always call the Rollout API to get this data as well).
Here is how you would modify the previous code:
import React, { useState, useEffect } from "react";
import { RolloutLinkProvider, CredentialsManager } from "@rollout/link-react";
function fetchRolloutToken() {
// We generate the token on the server in order to keep
// the ROLLOUT_CLIENT_SECRET secure.
return fetch("/rollout-token")
.then((resp) => resp.json())
.then((data) => data.token);
}
export function App() {
// This handler is called when new credentials are added
// Example callback data:
// id: "cred_12345abcde" - unique identifier for the credential
// appKey: "follow-up-boss" - identifies the integration type
const handleCredentialAdded = async ({ id, appKey }) => {
// Log the credential details
console.log(`New credential added - ID: ${id}, App: ${appKey}`);
// Here you could make an API call to your backend to store
// or change UI state for your application
};
return (
<div id="app">
<RolloutLinkProvider token={fetchRolloutToken}>
<CredentialsManager onCredentialAdded={handleCredentialAdded} />
</RolloutLinkProvider>
</div>
);
}